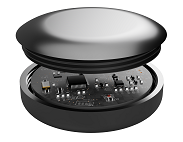 |
Master Thesis
V1.0
Research and Design of Sensor Node for NMSD Treatment
|
Go to the documentation of this file.
17 #ifndef ICM_20948_ICM20948_H_
18 #define ICM_20948_ICM20948_H_
uint32_t ICM_20948_gyroCalibrate(float *gyroBiasScaled)
Gyroscope calibration function. Reads the gyroscope values while the device is at rest and in level....
void ICM_20948_chipSelectSet(bool enable)
Set chipselect pin for SPI functionality.
void ICM_20948_set_mag_transfer(bool read)
Set magnetometer data transfer on integrated I2C controller inside ICM20948.
uint32_t ICM_20948_sensorEnable(bool accel, bool gyro, bool temp)
Enable selected sensors.
float ICM_20948_accelSampleRateSet(float sampleRate)
Sets the accelerometer sample rate.
void ICM_20948_read_mag_register(uint8_t addr, uint8_t numBytes, uint8_t *data)
Read register in the AK09916 magnetometer device.
void ICM_20948_enable_SPI(bool enable)
Enables the necessary SPI interface to communicate with ICM20948.
uint32_t ICM_20948_wakeOnMotionITEnable(bool enable, uint8_t womThreshold, float sampleRate)
Set wake on motion interrupt.
void ICM_20948_Init()
Init function for ICM20948.
void ICM_20948_write_mag_register(uint8_t addr, uint8_t data)
Writes a uint8_t to the I2C address of the magnetometer of the ICM_20948.
void ICM_20948_Init2()
Second init function for ICM20948, use when waking from sleep.
uint32_t ICM_20948_reset_mag(void)
Reset magnetometer.
uint32_t ICM_20948_set_mag_mode(uint8_t magMode)
Set magnetometer mode (sample rate / on-off)
uint32_t ICM_20948_accelDataRead(float *accel)
Read RAW accelerometer data.
void ICM_20948_magn_to_angle(float *magn, float *angle)
Test function for non-tilt compensated compass.
uint32_t ICM_20948_gyroResolutionGet(float *gyroRes)
Set gyro resolution.
void ICM_20948_registerRead(uint16_t addr, int numBytes, uint8_t *data)
Read registers from IMU.
uint32_t ICM_20948_min_max_mag(int16_t *minMag, int16_t *maxMag)
Calculate minimum and maximum magnetometer values, which are used for calibration.
uint32_t ICM_20948_gyroDataRead(float *gyro)
Read gyroscope data from IMU.
uint32_t ICM_20948_reset(void)
Reset IMU.
void ICM_20948_write(uint16_t addr, uint8_t data)
uint32_t ICM_20948_accelBandwidthSet(uint8_t accelBw)
Set acceleromter bandwidth.
uint8_t ICM_20948_read(uint16_t addr)
Read single register from IMU using SPI.
float ICM_20948_gyroSampleRateSet(float sampleRate)
Sets the gyro sample rate.
uint32_t ICM_20948_sampleRateSet(float sampleRate)
Combination function to set sample rate of gyro and accel at once.
bool ICM_20948_calibrate_mag(float *offset, float *scale)
Magnetometer calibration function. Get magnetometer minimum and maximum values, while moving the devi...
uint32_t ICM_20948_interruptStatusRead(uint32_t *intStatus)
Read staus of interrupt IMU.
uint32_t ICM_20948_cycleModeEnable(bool enable)
Enable cycle mode on IMU.
void ICM_20948_registerWrite(uint16_t addr, uint8_t data)
Writes registers from IMU.
uint32_t ICM_20948_lowPowerModeEnter(bool enAccel, bool enGyro, bool enTemp)
Enter low power mode for selected sensors.
uint32_t ICM_20948_accelGyroCalibrate(float *accelBiasScaled, float *gyroBiasScaled)
Accelerometer and gyroscope calibration function. Reads the gyroscope and accelerometer values while ...
uint32_t ICM_20948_accelFullscaleSet(uint8_t accelFs)
Set accelerometer full scale range.
uint32_t ICM_20948_interruptEnable(bool dataReadyEnable, bool womEnable)
Enable interrupt for data ready or wake on motion.
void ICM_20948_bankSelect(uint8_t bank)
Select appropriate bank in ICM20948 based on first bits of address.
uint32_t ICM_20948_gyroBandwidthSet(uint8_t gyroBw)
Set gyroscope bandwidth.
void ICM_20948_magRawDataRead(float *raw_magn)
Read magnetometer raw values.
void ICM_20948_Init_SPI()
Initiate SPI functionality for ICM20948.
void ICM_20948_magDataRead(float *magn)
Convert raw magnetometer values to calibrated and scaled ones.
uint32_t ICM_20948_accelResolutionGet(float *accelRes)
Get accelerometer resultion.
uint32_t ICM_20948_latchEnable(bool enable)
Enables / disables the 50 micro seconds interrupt pin functionality.
uint32_t ICM_20948_sleepModeEnable(bool enable)
Sleep mode enable function.
void ICM_20948_power(bool enable)
Turns the GPIO pin high to provide power to the ICM20948.
void ICM_20948_printAllData()
Print all gyro + accel + magn data using dbprint.
uint32_t ICM_20948_gyroFullscaleSet(uint8_t gyroFs)
Set gyroscope full scale range.