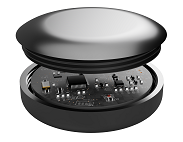 |
Master Thesis
V1.0
Research and Design of Sensor Node for NMSD Treatment
|
Go to the documentation of this file.
59 #include "em_system.h"
60 #include "em_device.h"
69 #include "rtcdriver.h"
111 #include "bsp_trace.h"
172 if( mean_gyro < 0.1 )
238 #if DEBUG_DBPRINT == 0
278 #if DEBUG_DBPRINT == 0
286 GPIO_PinOutSet(gpioPortE, 11);
287 GPIO_PinOutClear(gpioPortE, 11);
347 CMU_ClockSelectSet(cmuClock_HF, cmuSelect_HFXO);
350 CMU_ClockEnable(cmuClock_HFPER,
true);
351 CMU_ClockEnable(cmuClock_GPIO,
true);
355 GPIO_PinModeSet(gpioPortE, 11, gpioModePushPull, 0);
371 #if DEBUG_DBPRINT == 1
393 #if DEBUG_DBPRINT == 1
398 #if DEBUG_DBPRINT == 0
409 #if DEBUG_DBPRINT == 0
425 for(uint8_t i=0; i<8; i++)
450 #if DEBUG_DBPRINTs == 1
456 #if DEBUG_DBPRINTs == 1
470 #if DEBUG_DBPRINTs == 1
472 dbprintInt((
int) (ICM_20948_euler_angles[0] *100) );
475 dbprintInt((
int) (ICM_20948_euler_angles[1] *100) );
481 #if DEBUG_DBPRINTs == 1
503 #if DEBUG_DBPRINT == 1
525 CMU_ClockEnable(cmuClock_ADC0,
false);
528 GPIO_PinModeSet(gpioPortE, 11, gpioModeDisabled, 0);
557 CMU_ClockSelectSet(cmuClock_HF, cmuSelect_HFXO);
562 CMU_ClockEnable(cmuClock_ADC0,
true);
580 GPIO_PinModeSet(gpioPortE, 11, gpioModePushPull, 0);
584 #if DEBUG_DBPRINT == 1
592 #if DEBUG_DBPRINT == 1
621 GPIO_IntClear(0x5555);
622 #if DEBUG_DBPRINTs == 1
639 if(appState !=
SLEEP)
654 GPIO_IntClear(0xAAAA);
656 #if DEBUG_DBPRINTs == 1
void CheckIMUidle(void)
Function called by RTC timer every second.
void dbprint_INIT(USART_TypeDef *pointer, uint8_t location, bool vcom, bool interrupts)
void BLE_sendData(uint8_t *data, uint8_t *batt, uint8_t length, uint8_t *ble_data)
Send all the data over BLE.
uint32_t ICM_20948_accelDataRead(float *accel)
Read RAW accelerometer data.
uint32_t ICM_20948_lowPowerModeEnter(bool enAccel, bool enGyro, bool enTemp)
Enter low power mode for selected sensors.
uint8_t BLE_data[sizeof(float) *3+5]
uint32_t interruptStatus[1]
RTCDRV_TimerID_t IMU_Idle_Timer
void BLE_power(bool enable)
Turn power on/off for BLE module.
uint8_t ble_sec_not_connected
uint32_t ICM_20948_wakeOnMotionITEnable(bool enable, uint8_t womThreshold, float sampleRate)
Set wake on motion interrupt.
void dbprint(char *message)
void delay(uint32_t msDelay)
Wait for a certain amount of milliseconds in EM2/3.
void dbprintInt(int32_t value)
Advanced funcions to control and read data from the ICM-20948.
void BLE_rxtx_enable(bool enable)
Enable disable RX/TX pins from BLE module.
enum app_states APP_State_t
void BLE_check_connect()
Check if LED pin on BLE is high to check if the module is connected to a receiver.
UART communication with BLE.
Code to detect and generate interrupts.
void blink(uint8_t times)
Function to blink LED on sensor node.
void ICM_20948_Init()
Init function for ICM20948.
void initADC(void)
Initialisation of the ADC.
void dbprintlnInt(int32_t value)
void IIC_Enable(bool enable)
uint32_t ICM_20948_gyroDataRead(float *gyro)
Read gyroscope data from IMU.
void measure_send(void)
Function called by interrupt from IMU @50 Hz.
millis and micros functionality
void ADC_Batt_Read(void)
Do single ADC conversion (read)
void BLE_connect()
Connect sequence BLE.
void ICM_20948_Init2()
Second init function for ICM20948, use when waking from sleep.
uint32_t ICM_20948_interruptEnable(bool dataReadyEnable, bool womEnable)
Enable interrupt for data ready or wake on motion.
void ICM_20948_read_mag_register(uint8_t addr, uint8_t numBytes, uint8_t *data)
Read register in the AK09916 magnetometer device.
uint32_t ICM_20948_set_mag_mode(uint8_t magMode)
Set magnetometer mode (sample rate / on-off)
bool ICM_20948_calibrate_mag(float *offset, float *scale)
Magnetometer calibration function. Get magnetometer minimum and maximum values, while moving the devi...
void ADC_get_batt(uint8_t *percent)
Get battery in percentage.
void GPIO_EVEN_IRQHandler(void)
GPIO Even IRQ for pushbuttons on even-numbered pins.
void initGPIO_interrupt(void)
GPIO interrupt initialization.
File to keep track of most the pins used, pins for BLE are in own file BLE.h.
int main(void)
Main function.
float ICM_20948_euler_angles[3]
#define AK09916_BIT_MODE_POWER_DOWN
void ICM_20948_magDataRead(float *magn)
Convert raw magnetometer values to calibrated and scaled ones.
uint32_t ICM_20948_accelGyroCalibrate(float *accelBiasScaled, float *gyroBiasScaled)
Accelerometer and gyroscope calibration function. Reads the gyroscope and accelerometer values while ...
void GPIO_ODD_IRQHandler(void)
GPIO Odd IRQ for pushbuttons on odd-numbered pins.
void QuaternionsToEulerAngles(float *euler_angles)
Convert quaternions to euler angles.
void float_to_uint8_t_x3(float *in, uint8_t *out)
Float to uint8_t conversion x3.
uint8_t BLE_euler_angles[sizeof(float) *3]
void dbprintln(char *message)
void MadgwickAHRSupdate(float gx, float gy, float gz, float ax, float ay, float az, float mx, float my, float mz)
Madgwick algorithm.
Enable or disable printing to UART with dbprint.
void BLE_disconnect()
Disconnect BLE from receiver.
void BLE_Init()
BLE chip init.
Send data via BLE wirelessly.
I2C function to read-write the I2C bus, based on DRAMCO code.