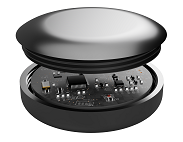 |
Master Thesis
V1.0
Research and Design of Sensor Node for NMSD Treatment
|
Go to the documentation of this file.
18 #include "em_device.h"
35 #include "rtcdriver.h"
41 #define M_PI 3.14159265358979323846
42 int32_t mag_minimumRange = 40;
53 int16_t
tX[3] = {1, 0, 0};
54 int16_t
tY[3] = {0, -1, 0};
55 int16_t
tZ[3] = {0, 0, -1};
66 static float _hxb = 0, _hyb = 0, _hzb = 0;
67 static float _hxs = 1.0f, _hys = 1.0f,_hzs = 1.0f;
88 CMU_ClockEnable(cmuClock_HFPER,
true);
89 CMU_ClockEnable(cmuClock_GPIO,
true);
192 #if DEBUG_DBPRINT == 1
193 dbprint(
"ICM20948 WHOAMI OKE");
241 #if DEBUG_DBPRINT == 1
279 CMU_ClockEnable(cmuClock_HFPER,
true);
280 CMU_ClockEnable(cmuClock_GPIO,
true);
281 CMU_ClockEnable(cmuClock_USART0,
true);
293 USART_InitSync_TypeDef config = USART_INITSYNC_DEFAULT;
294 config.master =
true;
296 config.autoCsEnable =
true;
297 config.clockMode = usartClockMode0;
299 config.enable = usartDisable;
302 config.clockMode = usartClockMode0;
304 config.baudrate = 4000000;
305 config.databits = usartDatabits8;
306 config.autoTx =
false;
307 config.autoCsEnable =
false;
310 USART_InitSync(USART0, &config);
313 USART0->ROUTE = USART_ROUTE_CLKPEN | USART_ROUTE_CSPEN | USART_ROUTE_TXPEN | USART_ROUTE_RXPEN | USART_ROUTE_LOCATION_LOC0;
316 USART_Enable(USART0, usartEnable);
320 GPIO_PinOutSet(gpioPortE, 13);
349 CMU_ClockEnable(cmuClock_USART0,
true);
351 USART_Enable(USART0, usartEnable);
365 CMU_ClockEnable(cmuClock_USART0,
false);
367 USART_Enable(USART0, usartDisable);
428 #if DEBUG_DBPRINTs == 1
462 while( WhoAmI != 0xEA )
532 wBuffer[1] = (bank << 4);
554 reg_address = (uint8_t) (addr & 0b01111111);
556 bank = (uint8_t) (addr >> 7);
567 USART_SpiTransfer(
SPI, (reg_address | 0b10000000));
570 data = USART_SpiTransfer(
SPI, 0x00);
661 uint8_t rLength = (uint8_t) numBytes;
664 regAddr = (uint8_t) (addr & 0x7F);
665 bank = (uint8_t) (addr >> 7);
670 wBuffer[0] = regAddr;
702 regAddr = (uint8_t) (addr & 0x7F);
703 bank = (uint8_t) (addr >> 7);
708 wBuffer[0] = regAddr;
796 temp = ( (int16_t) rawData[0] << 8) | rawData[1];
797 gyro[0] = (float) temp * gyroRes;
798 temp = ( (int16_t) rawData[2] << 8) | rawData[3];
799 gyro[1] = (float) temp * gyroRes;
800 temp = ( (int16_t) rawData[4] << 8) | rawData[5];
801 gyro[2] = (float) temp * gyroRes;
828 *gyroRes = 250.0 / 32768.0;
832 *gyroRes = 500.0 / 32768.0;
836 *gyroRes = 1000.0 / 32768.0;
840 *gyroRes = 2000.0 / 32768.0;
862 *intStatus = (uint32_t) reg[0];
863 *intStatus |= ( ( (uint32_t) reg[1]) << 8);
864 *intStatus |= ( ( (uint32_t) reg[2]) << 16);
865 *intStatus |= ( ( (uint32_t) reg[3]) << 24);
902 if ( dataReadyEnable ) {
950 float gyroSampleRate;
953 gyroSampleRate = (1125.0 / sampleRate) - 1.0;
956 if ( gyroSampleRate > 255.0 ) {
957 gyroSampleRate = 255.0;
960 if ( gyroSampleRate < 0 ) {
961 gyroSampleRate = 0.0;
965 gyroDiv = (uint8_t) gyroSampleRate;
969 gyroSampleRate = 1125.0 / (gyroDiv + 1);
971 return gyroSampleRate;
991 float accelSampleRate;
994 accelSampleRate = (1125.0 / sampleRate) - 1.0;
997 if ( accelSampleRate > 4095.0 ) {
998 accelSampleRate = 4095.0;
1001 if ( accelSampleRate < 0 ) {
1002 accelSampleRate = 0.0;
1006 accelDiv = (uint16_t) accelSampleRate;
1011 accelSampleRate = 1125.0 / (accelDiv + 1);
1013 return accelSampleRate;
1043 if ( enAccel || enGyro || enTemp ) {
1097 uint8_t pwrManagement1;
1098 uint8_t pwrManagement2;
1183 temp = ( (int16_t) rawData[0] << 8) | rawData[1];
1184 accel[0] = (float) temp * accelRes;
1185 temp = ( (int16_t) rawData[2] << 8) | rawData[3];
1186 accel[1] = (float) temp * accelRes;
1187 temp = ( (int16_t) rawData[4] << 8) | rawData[5];
1188 accel[2] = (float) temp * accelRes;
1213 *accelRes = 2.0 / 32768.0;
1217 *accelRes = 4.0 / 32768.0;
1221 *accelRes = 8.0 / 32768.0;
1225 *accelRes = 16.0 / 32768.0;
1447 bool read_old = !read;
1450 if (read != read_old) {
1480 #if DEBUG_DBPRINT == 1
1481 dbprint(
"Failed to communicate with compass: NACK");
1486 #if DEBUG_DBPRINT == 1
1671 switch ( magMode ) {
1722 #if DEBUG_DBPRINTs == 1
1795 angle[0] = atan2(magn[1], magn[0]) *
M_PI/180.0f;
1823 uint16_t i, packetCount, fifoCount;
1824 int32_t gyroBias[3] = { 0, 0, 0 };
1825 int32_t accelBias[3] = { 0, 0, 0 };
1826 int32_t accelTemp[3];
1827 int32_t gyroTemp[3];
1828 int32_t accelBiasFactory[3];
1829 int32_t gyroBiasStored[3];
1830 float gyroRes, accelRes;
1872 while ( fifoCount < 4080 ) {
1877 fifoCount = ( (uint16_t) (
data[0] << 8) |
data[1]);
1887 fifoCount = ( (uint16_t) (
data[0] << 8) |
data[1]);
1890 packetCount = fifoCount / 12;
1893 for ( i = 0; i < packetCount; i++ ) {
1896 accelTemp[0] = ( (int16_t) (
data[0] << 8) |
data[1]);
1897 accelTemp[1] = ( (int16_t) (
data[2] << 8) |
data[3]);
1898 accelTemp[2] = ( (int16_t) (
data[4] << 8) |
data[5]);
1899 gyroTemp[0] = ( (int16_t) (
data[6] << 8) |
data[7]);
1900 gyroTemp[1] = ( (int16_t) (
data[8] << 8) |
data[9]);
1901 gyroTemp[2] = ( (int16_t) (
data[10] << 8) |
data[11]);
1904 accelBias[0] += accelTemp[0];
1905 accelBias[1] += accelTemp[1];
1906 accelBias[2] += accelTemp[2];
1907 gyroBias[0] += gyroTemp[0];
1908 gyroBias[1] += gyroTemp[1];
1909 gyroBias[2] += gyroTemp[2];
1913 accelBias[0] /= packetCount;
1914 accelBias[1] /= packetCount;
1915 accelBias[2] /= packetCount;
1916 gyroBias[0] /= packetCount;
1917 gyroBias[1] /= packetCount;
1918 gyroBias[2] /= packetCount;
1921 if ( accelBias[2] > 0L ) {
1922 accelBias[2] -= (int32_t) (1.0 / accelRes);
1924 accelBias[2] += (int32_t) (1.0 / accelRes);
1928 gyroBiasScaled[0] = (float) gyroBias[0] * gyroRes;
1929 gyroBiasScaled[1] = (float) gyroBias[1] * gyroRes;
1930 gyroBiasScaled[2] = (float) gyroBias[2] * gyroRes;
1934 gyroBiasStored[0] = ( (int16_t) (
data[0] << 8) |
data[1]);
1936 gyroBiasStored[1] = ( (int16_t) (
data[0] << 8) |
data[1]);
1938 gyroBiasStored[2] = ( (int16_t) (
data[0] << 8) |
data[1]);
1943 gyroBiasStored[0] -= gyroBias[0] / 4;
1944 gyroBiasStored[1] -= gyroBias[1] / 4;
1945 gyroBiasStored[2] -= gyroBias[2] / 4;
1948 data[0] = (gyroBiasStored[0] >> 8) & 0xFF;
1949 data[1] = (gyroBiasStored[0]) & 0xFF;
1950 data[2] = (gyroBiasStored[1] >> 8) & 0xFF;
1951 data[3] = (gyroBiasStored[1]) & 0xFF;
1952 data[4] = (gyroBiasStored[2] >> 8) & 0xFF;
1953 data[5] = (gyroBiasStored[2]) & 0xFF;
1971 accelBiasFactory[0] = ( (int16_t) (
data[0] << 8) |
data[1]);
1973 accelBiasFactory[1] = ( (int16_t) (
data[0] << 8) |
data[1]);
1975 accelBiasFactory[2] = ( (int16_t) (
data[0] << 8) |
data[1]);
1982 accelBiasFactory[0] -= ( (accelBias[0] / 8) & ~1);
1983 accelBiasFactory[1] -= ( (accelBias[1] / 8) & ~1);
1984 accelBiasFactory[2] -= ( (accelBias[2] / 8) & ~1);
1987 data[0] = (accelBiasFactory[0] >> 8) & 0xFF;
1988 data[1] = (accelBiasFactory[0]) & 0xFF;
1989 data[2] = (accelBiasFactory[1] >> 8) & 0xFF;
1990 data[3] = (accelBiasFactory[1]) & 0xFF;
1991 data[4] = (accelBiasFactory[2] >> 8) & 0xFF;
1992 data[5] = (accelBiasFactory[2]) & 0xFF;
2003 accelBiasScaled[0] = (float) accelBias[0] * accelRes;
2004 accelBiasScaled[1] = (float) accelBias[1] * accelRes;
2005 accelBiasScaled[2] = (float) accelBias[2] * accelRes;
2034 uint16_t i, packetCount, fifoCount;
2035 int32_t gyroBias[3] = { 0, 0, 0 };
2036 int32_t gyroTemp[3];
2037 int32_t gyroBiasStored[3];
2077 while ( fifoCount < 4080 ) {
2084 fifoCount = ( (uint16_t) (
data[0] << 8) |
data[1]);
2094 fifoCount = ( (uint16_t) (
data[0] << 8) |
data[1]);
2097 packetCount = fifoCount / 12;
2100 for ( i = 0; i < packetCount; i++ ) {
2103 gyroTemp[0] = ( (int16_t) (
data[6] << 8) |
data[7]);
2104 gyroTemp[1] = ( (int16_t) (
data[8] << 8) |
data[9]);
2105 gyroTemp[2] = ( (int16_t) (
data[10] << 8) |
data[11]);
2108 gyroBias[0] += gyroTemp[0];
2109 gyroBias[1] += gyroTemp[1];
2110 gyroBias[2] += gyroTemp[2];
2114 gyroBias[0] /= packetCount;
2115 gyroBias[1] /= packetCount;
2116 gyroBias[2] /= packetCount;
2119 gyroBiasScaled[0] = (float) gyroBias[0] * gyroRes;
2120 gyroBiasScaled[1] = (float) gyroBias[1] * gyroRes;
2121 gyroBiasScaled[2] = (float) gyroBias[2] * gyroRes;
2125 gyroBiasStored[0] = ( (int16_t) (
data[0] << 8) |
data[1]);
2128 gyroBiasStored[1] = ( (int16_t) (
data[0] << 8) |
data[1]);
2131 gyroBiasStored[2] = ( (int16_t) (
data[0] << 8) |
data[1]);
2136 gyroBiasStored[0] -= gyroBias[0] / 4;
2137 gyroBiasStored[1] -= gyroBias[1] / 4;
2138 gyroBiasStored[2] -= gyroBias[2] / 4;
2141 data[0] = (gyroBiasStored[0] >> 8) & 0xFF;
2142 data[1] = (gyroBiasStored[0]) & 0xFF;
2143 data[2] = (gyroBiasStored[1] >> 8) & 0xFF;
2144 data[3] = (gyroBiasStored[1]) & 0xFF;
2145 data[4] = (gyroBiasStored[2] >> 8) & 0xFF;
2146 data[5] = (gyroBiasStored[2]) & 0xFF;
2197 uint32_t max_time = 15000;
2198 uint32_t t_start =
millis();
2201 bool miniumRange_mx =
false;
2202 bool miniumRange_my =
false;
2203 bool miniumRange_mz =
false;
2206 while (!miniumRange_mx || !miniumRange_my || !miniumRange_mz) {
2207 while ((
millis() - t_start) < max_time) {
2212 if (m[0] < minMag[0]) {
2215 if (m[0] > maxMag[0]) {
2219 if (m[1] < minMag[1]) {
2222 if (m[1] > maxMag[1]) {
2226 if (m[2] < minMag[2]) {
2229 if (m[2] > maxMag[2]) {
2234 miniumRange_mx =
true;
2237 miniumRange_my =
true;
2240 miniumRange_mz =
true;
2246 #if DEBUG_DBPRINT == 1
2292 int32_t sum_mx, sum_my, sum_mz;
2293 int32_t dif_mx, dif_my, dif_mz, dif_m;
2307 sum_mx = (int32_t) maxMag[0] + minMag[0];
2308 sum_my = (int32_t) maxMag[1] + minMag[1];
2309 sum_mz = (int32_t) maxMag[2] + minMag[2];
2311 dif_mx = (int32_t) maxMag[0] - minMag[0];
2312 dif_my = (int32_t) maxMag[1] - minMag[1];
2313 dif_mz = (int32_t) maxMag[2] - minMag[2];
2315 offset[0] = -0.5f * sum_mx;
2316 offset[1] = -0.5f * sum_my;
2317 offset[2] = -0.5f * sum_mz;
2319 dif_m = (dif_mx + dif_my + dif_mz) / 3;
2321 scale[0] = (float) dif_m / dif_mx;
2322 scale[1] = (float) dif_m / dif_my;
2323 scale[2] = (float) dif_m / dif_mz;
#define ICM_20948_REG_FIFO_MODE
#define ICM_20948_CLK_PORT
#define ICM_20948_REG_PWR_MGMT_2
#define ICM_20948_REG_INT_ENABLE_1
#define ICM_20948_BIT_SLEEP
#define ICM_20948_ACCEL_FULLSCALE_8G
uint32_t ICM_20948_gyroBandwidthSet(uint8_t gyroBw)
Set gyroscope bandwidth.
void ICM_20948_magRawDataRead(float *raw_magn)
Read magnetometer raw values.
#define ICM_20948_MOSI_PORT
#define ICM_20948_BIT_LP_EN
#define ICM_20948_GYRO_FULLSCALE_1000DPS
void ICM_20948_chipSelectSet(bool enable)
Set chipselect pin for SPI functionality.
#define ICM_20948_GYRO_FULLSCALE_2000DPS
#define ICM_20948_MASK_ACCEL_FULLSCALE
uint32_t ICM_20948_accelResolutionGet(float *accelRes)
Get accelerometer resultion.
#define ICM_20948_REG_ACCEL_WOM_THR
#define ICM20948_DEVICE_ID
#define ICM_20948_REG_FIFO_COUNT_H
uint32_t ICM_20948_latchEnable(bool enable)
Enables / disables the 50 micro seconds interrupt pin functionality.
#define ICM_20948_ACCEL_BW_246HZ
void ICM_20948_enable_SPI(bool enable)
Enables the necessary SPI interface to communicate with ICM20948.
#define AK09916_REG_WHO_AM_I
uint32_t ICM_20948_accelDataRead(float *accel)
Read RAW accelerometer data.
#define AK09916_BIT_I2C_SLV_ADDR
void ICM_20948_write_mag_register(uint8_t addr, uint8_t data)
Writes a uint8_t to the I2C address of the magnetometer of the ICM_20948.
uint32_t ICM_20948_lowPowerModeEnter(bool enAccel, bool enGyro, bool enTemp)
Enter low power mode for selected sensors.
#define ICM_20948_POWER_PORT
#define ICM_20948_POWER_PIN
uint32_t ICM_20948_accelFullscaleSet(uint8_t accelFs)
Set accelerometer full scale range.
void ICM_20948_set_mag_transfer(bool read)
Set magnetometer data transfer on integrated I2C controller inside ICM20948.
#define ICM_20948_MISO_PIN
void ICM_20948_magn_to_angle(float *magn, float *angle)
Test function for non-tilt compensated compass.
bool ICM_20948_Initialized
#define ICM_20948_BIT_RAW_DATA_0_RDY_EN
#define ICM_20948_REG_ZG_OFFS_USRH
void waitForSlave4()
Wait for I2C slave (internal I2C controller on ICM20948)
#define AK09916_MODE_50HZ
uint32_t ICM_20948_gyroCalibrate(float *gyroBiasScaled)
Gyroscope calibration function. Reads the gyroscope values while the device is at rest and in level....
#define AK09916_MODE_20HZ
uint32_t ICM_20948_wakeOnMotionITEnable(bool enable, uint8_t womThreshold, float sampleRate)
Set wake on motion interrupt.
#define ICM_20948_REG_FIFO_RST
void dbprint(char *message)
#define ICM_20948_REG_XG_OFFS_USRL
void delay(uint32_t msDelay)
Wait for a certain amount of milliseconds in EM2/3.
#define ICM_20948_REG_I2C_SLV4_DI
#define ICM_20948_BIT_WOM_INT_EN
void dbprintInt(int32_t value)
#define ICM_20948_BIT_GYRO_CYCLE
Advanced funcions to control and read data from the ICM-20948.
#define ICM_20948_BIT_TEMP_DIS
#define ICM_20948_I2C_ADDRESS
#define ICM_20948_BIT_PWR_GYRO_STBY
#define ICM_20948_REG_XG_OFFS_USRH
uint32_t ICM_20948_sensorEnable(bool accel, bool gyro, bool temp)
Enable selected sensors.
float ICM_20948_accelSampleRateSet(float sampleRate)
Sets the accelerometer sample rate.
uint32_t millis(void)
Millis Arduino like functionality for EFM32HG.
#define ICM_20948_REG_YG_OFFS_USRL
#define ICM_20948_REG_USER_CTRL
#define ICM_20948_REG_YA_OFFSET_H
#define ICM_20948_BIT_ACCEL_CYCLE
#define ICM_20948_GYRO_BW_12HZ
#define ICM_20948_ACCEL_BW_1210HZ
#define ICM_20948_ACCEL_FULLSCALE_4G
#define ICM_20948_REG_INT_ENABLE
#define ICM_20948_REG_BANK_SEL
uint32_t ICM_20948_min_max_mag(int16_t *minMag, int16_t *maxMag)
Calculate minimum and maximum magnetometer values, which are used for calibration.
#define AK09916_REG_CONTROL_3
#define ICM_20948_MOSI_PIN
#define AK09916_MODE_100HZ
uint32_t ICM_20948_sampleRateSet(float sampleRate)
Combination function to set sample rate of gyro and accel at once.
#define ICM_20948_GYRO_FULLSCALE_500DPS
#define ICM_20948_BIT_ACCEL_FIFO_EN
#define ICM_20948_REG_FIFO_R_W
#define ICM_20948_REG_XA_OFFSET_L
void ICM_20948_Init()
Init function for ICM20948.
#define ICM_20948_BIT_H_RESET
#define ICM_20948_REG_I2C_SLV4_DO
void dbprintlnInt(int32_t value)
uint32_t ICM_20948_gyroDataRead(float *gyro)
Read gyroscope data from IMU.
#define ICM_20948_REG_GYRO_SMPLRT_DIV
#define ICM_20948_REG_ACCEL_SMPLRT_DIV_2
void IIC_Init(void)
Setup I2C functionality.
#define ICM_20948_REG_I2C_SLV0_ADDR
millis and micros functionality
#define ICM_20948_REG_ACCEL_CONFIG
bool IIC_WriteBuffer(uint8_t iicAddress, uint8_t *wBuffer, uint8_t wLength)
I2C write functionality.
#define AK09916_MODE_SINGLE
uint32_t ICM_20948_accelBandwidthSet(uint8_t accelBw)
Set acceleromter bandwidth.
#define ICM_20948_REG_YG_OFFS_USRH
#define ICM_20948_BIT_SLV4_NACK
void ICM_20948_Init2()
Second init function for ICM20948, use when waking from sleep.
#define ICM_20948_MISO_PORT
#define ICM_20948_BIT_I2C_SLV_EN
uint32_t ICM_20948_interruptEnable(bool dataReadyEnable, bool womEnable)
Enable interrupt for data ready or wake on motion.
uint32_t ICM_20948_reset_mag(void)
Reset magnetometer.
uint32_t ICM_20948_cycleModeEnable(bool enable)
Enable cycle mode on IMU.
unsigned char readMagRegister(unsigned char magreg)
Read magnetometer register from I2C controller in ICM20948.
uint8_t ICM_20948_read(uint16_t addr)
Read single register from IMU using SPI.
void ICM_20948_read_mag_register(uint8_t addr, uint8_t numBytes, uint8_t *data)
Read register in the AK09916 magnetometer device.
#define ICM_20948_MASK_GYRO_FULLSCALE
#define ICM_20948_BIT_FIFO_EN
float ICM_20948_gyroSampleRateSet(float sampleRate)
Sets the gyro sample rate.
uint32_t ICM_20948_set_mag_mode(uint8_t magMode)
Set magnetometer mode (sample rate / on-off)
#define ICM_20948_MASK_GYRO_BW
bool ICM_20948_calibrate_mag(float *offset, float *scale)
Magnetometer calibration function. Get magnetometer minimum and maximum values, while moving the devi...
#define ICM_20948_ACCEL_FULLSCALE_2G
#define ICM_20948_BIT_I2C_READ
#define ICM_20948_REG_LP_CONFIG
#define ICM_20948_CS_PORT
#define ICM_20948_REG_ZA_OFFSET_L
#define ICM_20948_REG_ZA_OFFSET_H
File to keep track of most the pins used, pins for BLE are in own file BLE.h.
void ICM_20948_registerRead(uint16_t addr, int numBytes, uint8_t *data)
Read registers from IMU.
#define AK09916_DEVICE_ID
#define AK09916_BIT_MODE_POWER_DOWN
void ICM_20948_Init_SPI()
Initiate SPI functionality for ICM20948.
#define ICM_20948_REG_YA_OFFSET_L
#define ICM_20948_REG_I2C_SLV4_CTRL
void ICM_20948_magDataRead(float *magn)
Convert raw magnetometer values to calibrated and scaled ones.
#define ICM_20948_BIT_CLK_PLL
void ICM_20948_power(bool enable)
Turns the GPIO pin high to provide power to the ICM20948.
uint32_t ICM_20948_accelGyroCalibrate(float *accelBiasScaled, float *gyroBiasScaled)
Accelerometer and gyroscope calibration function. Reads the gyroscope and accelerometer values while ...
#define ICM_20948_REG_ZG_OFFS_USRL
#define ICM_20948_REG_INT_STATUS
uint32_t ICM_20948_interruptStatusRead(uint32_t *intStatus)
Read staus of interrupt IMU.
#define ICM_20948_BIT_ACCEL_INTEL_EN
#define ICM_20948_REG_ACCEL_XOUT_H_SH
uint32_t ICM_20948_sleepModeEnable(bool enable)
Sleep mode enable function.
uint32_t ICM_20948_reset(void)
Reset IMU.
void ICM_20948_bankSelect(uint8_t bank)
Select appropriate bank in ICM20948 based on first bits of address.
#define ICM_20948_BIT_ACCEL_INTEL_MODE
#define AK09916_MODE_10HZ
void writeMagRegister(unsigned char magreg, unsigned char val)
Write magnetometer register using internal I2C interface on ICM20948.
#define ICM_20948_BIT_PWR_ACCEL_STBY
#define ICM_20948_REG_INT_PIN_CFG
#define ICM_20948_REG_ACCEL_SMPLRT_DIV_1
#define ICM_20948_REG_WHO_AM_I
#define ICM_20948_BIT_I2C_SLV_READ
#define ICM_20948_ACCEL_FULLSCALE_16G
#define ICM_20948_REG_XA_OFFSET_H
#define AK09916_REG_CONTROL_2
uint32_t ICM_20948_gyroResolutionGet(float *gyroRes)
Set gyro resolution.
bool IIC_WriteReadBuffer(uint8_t iicAddress, uint8_t *wBuffer, uint8_t wLength, uint8_t *rBuffer, uint8_t rLength)
I2C read/write functionality, to read and write at the same time.
#define ICM_20948_REG_GYRO_CONFIG_1
uint32_t ICM_20948_gyroFullscaleSet(uint8_t gyroFs)
Set gyroscope full scale range.
#define ICM_20948_REG_PWR_MGMT_1
#define ICM_20948_MASK_ACCEL_BW
void ICM_20948_check_WhoAmI()
Check WhoAmI register of ICM20948.
#define ICM_20948_BITS_GYRO_FIFO_EN
void ICM_20948_printAllData()
Print all gyro + accel + magn data using dbprint.
void dbprintln(char *message)
#define ICM_20948_REG_I2C_SLV4_ADDR
void ICM_20948_registerWrite(uint16_t addr, uint8_t data)
Writes registers from IMU.
#define ICM_20948_REG_FIFO_EN_2
Enable or disable printing to UART with dbprint.
#define ICM_20948_REG_I2C_SLV4_REG
#define ICM_20948_CLK_PIN
#define ICM_20948_REG_ACCEL_INTEL_CTRL
#define ICM_20948_BIT_SLV4_DONE
#define ICM_20948_GYRO_FULLSCALE_250DPS
I2C function to read-write the I2C bus, based on DRAMCO code.
#define ICM_20948_REG_I2C_MST_STATUS